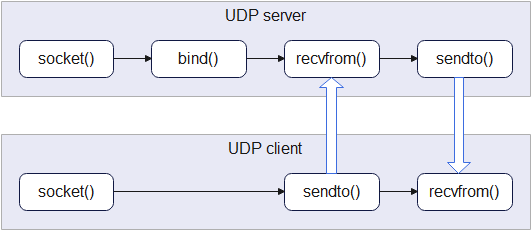
So far I have used a large number of custom functions to configure and control the WiFi networking, but before adding yet more functionality, I need to offer a simpler (and more standard) way of doing all this programming.
When it comes to network programming on Linux or Windows systems, there is only one widely-used Application Programming Interface (API), namely Network Sockets, also known as Internet Sockets. A socket is an endpoint on the network, defined by an IP address & port number; in previous parts, I have used sockets to construct DHCP & DNS clients, and now will use them in a general-purpose programming interface.
UDP & TCP, Client & Server
To recap on the difference between User Datagram Protocol (UDP) & Transmission Control Protocol (TCP), also Client & Server:
- UDP allows you to send blocks of data across the network, the maximum block size generally being around 1.5 kBytes. There is no synchronisation between sender & receiver, and no error handling, so there is no guarantee that the data will arrive at the destination.
- TCP starts with an exchange of packets to synchronise the two endpoints, and thereafter the transfer is bidirectional and stream-orientated; it acts like a transparent pipe between the sockets, carrying arbitrary numbers of bytes from one socket to the other. When the transfer is complete, either side can close the connection, but the closure will only be complete when both sides acknowledge it.
- A client initiates contact with a server, by sending UDP or TCP traffic to a well-known port on that system.
- A server takes possession of (‘binds to’) a specific UDP or TCP port number, and runs continuously, waiting for a client to initiate contact.
I’m starting with the simplest of these options, namely a UDP server.
UDP server
To create a server socket, it is just necessary to issue a socket() call, followed by bind() to take possession of a specific port, by providing an initialised structure. The same code applies for both Linux, and my RP2040 socket API:
#define PORTNUM 8080 // Server port number
#define MAXLEN 1024 // Maximum data length
int server_sock;
struct sockaddr_in server_addr, client_addr;
server_sock = socket(AF_INET, SOCK_DGRAM, 0);
if (server_sock < 0)
{
perror("socket creation failed");
return(1);
}
memset(&server_addr, 0, sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = INADDR_ANY;
server_addr.sin_port = htons(PORTNUM);
if (bind(server_sock, (struct sockaddr *)&server_addr, sizeof(server_addr)) < 0)
{
perror("bind failed");
return(1);
}
You may be surprised at the use of 2 structures, namely sockaddr_in, and sockaddr. The former is specific to Internet Protocol, whereas the latter is general-purpose, capable of being used with other network protocols. The structure has to be filled in with the address family (Internet Protocol) the desired IP address (zero for any), and the port number, byte-swapped to big-endian.
The code then enters an endless loop, waiting for a a datagram to be received, printing the data (on the assumption it is text), and returning a text string in response:
printf("UDP server on port %u\n", PORTNUM);
while (1)
{
memset(&client_addr, 0, sizeof(client_addr));
addrlen = sizeof(client_addr);
n = recvfrom(server_sock, buffer, MAXLEN-1, 0,
(struct sockaddr *)&client_addr, &addrlen);
if (n > 0)
{
buffer[n] = 0;
printf("Client %s: %s\n", inet_ntoa(client_addr.sin_addr), buffer);
n = sprintf(buffer, "Test %u\n", testnum++);
sendto(server_sock, buffer, n, MSG_CONFIRM,
(struct sockaddr *)&client_addr, addrlen);
}
}
It may seem strange to set ‘addrlen’ every time we go round the loop; this is for safety, as recvfrom() can potentially modify its value. Also, there is no guarantee that the incoming string is null-terminated, so a null is added at the end of the datagram, just in case.
Blocking
The above code ‘blocks’ on the receive function, that is to say it waits indefinitely until the data arrives, which is standard practice on Linux or Windows sockets – it is sensible when we have a multi-tasking operating system, but inconvenient if we are restricted to a single task, as the CPU can’t run any other code while waiting for incoming data.
There are various ways round this limitation, for example Linux has a ‘select’ function that allows you to poll the interface, checking if any incoming data is available. Alternatively, we can set a timeout on the socket read, for example 0.1 seconds (100,000 microseconds):
struct timeval tv;
tv.tv_sec = 0;
tv.tv_usec = 100000;
setsockopt(server_sock, SOL_SOCKET, SO_RCVTIMEO, &tv, sizeof(tv));
To demonstrate this functionality, we can add a useful feature whereby the LED is flashed every time an access to the server is made; if we set the timeout to 1000 microseconds, then the timeouts give us a convenient millisecond timer. The following code does a rapid flash of the LED until the unit joins the Wifi network, then a quick blink every 2 seconds, plus a flash every time the server is accessed.
sock_set_timeout(server_sock, 1000);
while (1)
{
memset(&client_addr, 0, sizeof(client_addr));
addrlen = sizeof(client_addr);
n = recvfrom(server_sock, buffer, MAXLEN, 0,
(struct sockaddr *)&client_addr, &addrlen);
if (n > 0)
{
buffer[n] = 0;
printf("Client %s: %s\n", inet_ntoa(client_addr.sin_addr), buffer);
n = sprintf(buffer, "Test %u\n", testnum++);
sendto(server_sock, buffer, n, 0, (struct sockaddr *)&client_addr, addrlen);
msec = 0;
}
else
{
msec++;
if (msec == 1)
wifi_set_led(1);
else if (msec == 100)
wifi_set_led(0);
else if (msec == 200 && !link_check())
msec = 0;
else if (msec == 2000)
msec = 0;
}
}
Test program
See the introduction for details on how to rebuild and program the udp_socket_server application into the Pico.
The simplest way to test the server is using the netcat application, that can easily be installed on the Raspberry Pi using ‘apt install’; you just need to note the IP address of the Pico as reported on the serial console, and enter it into the netcat command line, e.g. for an address of 192.168.1.240:
echo -n "hello" | nc -4u -w1 192.168.1.240 8080
The server will respond with an incrementing test number, e.g.
Test 1
Alternatively, here is a simple Python client program:
# Simple UDP client example
import time, socket
addr = "192.168.1.240", 8080
data = 'test'
while True:
client = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
client.settimeout(1)
client.sendto(str.encode(data), addr)
print("Tx: " + data);
try:
resp, server = client.recvfrom(1024)
except socket.timeout:
resp = None
print("Rx: " + "timeout" if not resp else resp.decode('utf-8'))
time.sleep(2)
# EOF
Or in C:
// Simple UDP client for Linux
#include <stdio.h>
#include <string.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <unistd.h>
#define SERVER_ADDR "192.168.1.240"
#define SERVER_PORT 8080
#define MAXLEN 1024
void sock_set_timeout(int sock, int usec);
int main(int argc, char **argv)
{
int sock, n;
struct sockaddr_in server_addr;
char txdata[MAXLEN] = "test", rxdata[MAXLEN];
sock = socket(AF_INET, SOCK_DGRAM, 0);
sock_set_timeout(sock, 100000);
bzero(&server_addr, sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = INADDR_ANY;
server_addr.sin_port = htons(SERVER_PORT);
while (1)
{
printf("Tx %s:%u: %s\n", SERVER_ADDR, SERVER_PORT, txdata);
sendto(sock, txdata, strlen(txdata), 0,
(struct sockaddr *)&server_addr, sizeof(server_addr));
n = recvfrom(sock, rxdata, MAXLEN-1, 0, NULL, NULL);
if (n < 0)
printf("Rx timeout\n");
else
{
rxdata[n] = 0;
printf("Rx %s\n", rxdata);
}
sleep(2);
}
return(0);
}
// Set socket timeout
void sock_set_timeout(int sock, int usec)
{
struct timeval tv;
tv.tv_sec = usec / 1000000;
tv.tv_usec = usec % 1000000;
setsockopt(sock, SOL_SOCKET, SO_RCVTIMEO, &tv, sizeof(tv));
}
Project links | |
---|---|
Introduction | Project overview |
Part 1 | Low-level interface; hardware & software |
Part 2 | Initialisation; CYW43xxx chip setup |
Part 3 | IOCTLs and events; driver communication |
Part 4 | Scan and join a network; WPA security |
Part 5 | ARP, IP and ICMP; IP addressing, and ping |
Part 6 | DHCP; fetching IP configuration from server |
Part 7 | DNS; domain name lookup |
Part 8 | UDP server socket |
Part 9 | TCP Web server |
Part 10 | Web camera |
Source code | Full C source code |
Copyright (c) Jeremy P Bentham 2022. Please credit this blog if you use the information or software in it.